How to access private Github repos when building Docker images?
If you landed on this page, it means you probably have the same issue I’ve stumbled upon as well: I need to access some private Github repos while building a docker image but I don’t want any ssh keys or credentials to end up in the final image.
While there’s already ways to achieve this, the recent additions of buildx (first release was back in April 2019) and experimental dockerfile syntaxes (available from v18.06
onwards) make it much easier to do it (or just more elegant).
Do note that you need to have Docker 18.09+
installed and you need to enable the experimental features for Docker (both for the CLI and the daemon).
To enable the experimental features for the CLI, you just need to add the following to your ~/.docker/config.json
config:
|
|
Preferences > Command Line
(see image below).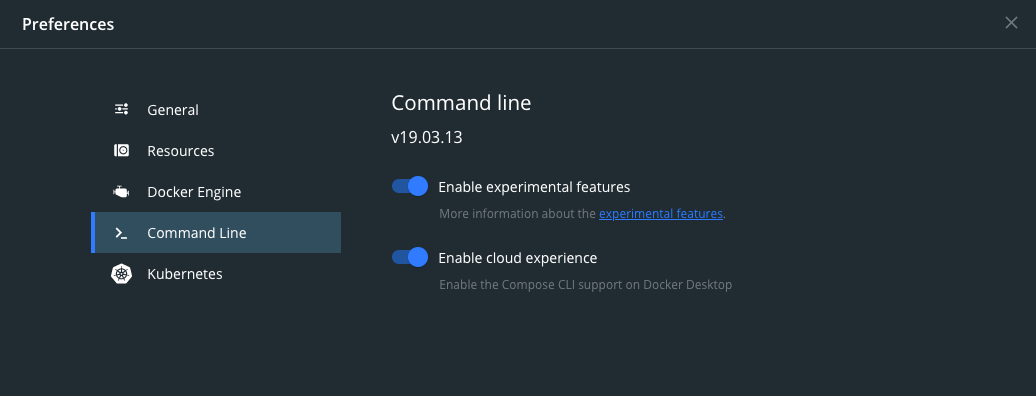
And to enable the experimental features for the daemon, you need to add the following to your /etc/docker/daemon.json
config:
|
|
~/.docker/daemon.json
. Or if you’re using the Desktop version, you’ll find these settings under Preferences > Docker Engine
(see image below).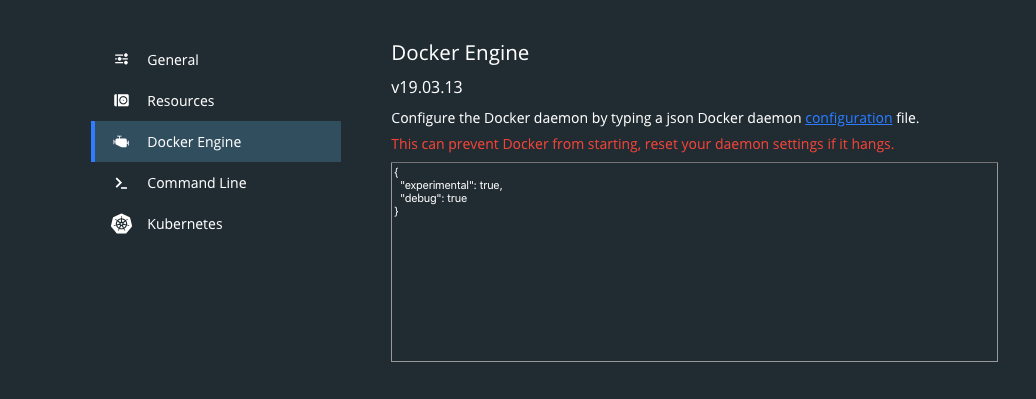
Furthermore, if you’re not using a Linux machine, you need to start the ssh-agent:
|
|
And add your current SSH key to the agent:
|
|
We also need to setup a known_hosts
file to avoid prompts from SSH when cloning:
|
|
Now create a dockerfile that just clones a private repo:
|
|
And build a base image off that which we’ll use in another image:
|
|
Lastly, create a dockerfile that copies whatever was in the private repo to the host:
|
|
And build the image to get the output:
|
|
If everything went well, you should see the private repo’s content under ./priv-code
.
Things to note:
- The above guide will only work for Github, though, with slight changes it can work for GitLab or others
- We’re using the secret feature to pass the
known_hosts
file to the base image, but we can easily run thessh-keyscan
just before thegit clone ...
- We’re configuring git to use SSH when cloning, but this might not work for everyone
- This guide has not been tested Windows
Finally, you can find this example at rolandjitsu/docker-ssh. The repo also includes examples of how to use the bake command and how a Github action that uses this approach would look like.